Using CakePHP in external PHP Systems (CMS's, Weblogs, etc.)
Posted on 4/5/06 by Felix Geisendörfer
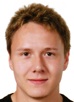
Deprecated post
The authors of this post have marked it as deprecated. This means the information displayed is most likely outdated, inaccurate, boring or a combination of all three.
Policy: We never delete deprecated posts, but they are not listed in our categories or show up in the search anymore.
Comments: You can continue to leave comments on this post, but please consult Google or our search first if you want to get an answer ; ).
Well CakePHP is a great PHP framework, in fact I think it is the best. But sometimes even that isn't good enough for keeping up with this really tight deadlines one might have. In those cases I tend to go with other OS systems that are already close to what I need. For now that's mostly been Drupal, but I'm also thinking about doing the same thing with WordPress on this site.
Now one might wonder what's the best way to utilize the powers of cakephp in those projects for getting the customization done as fast as possible. Now that I've tried a couple of approaches to do so (an earlier PoC can be seen here), mostly keeping the two systems in seperate folders and using customized .htaccess files to get the the two systems connected.
But today I started to work on a little bit on my Drake project again and found an even better way to go. With better I don't neccessary mean the "cakephp right way", but one that required very little code and is easy to maintain, which is my criteria.
I'll explain what I did for Drupal (and it should be just about 99% the same for any other system):
Where to put the foreign system?
Technically it would be a vendor, so one might think that's a good place to put it. But in most cases this won't work because parts of the foreign system will need to be accessible by the browser of the client. You could use .htaccess to do that, but you'd only make another folder useless that way, the webroot folder. And that's the place I decided to try out today.
Just take the whole foreign app (drupal for me) and paste it into cakephp's app/webroot folder. Now the other app is likely to already provide it's own .htacess as well as index.php. You should rename the cakephp index.php to cakephp.php before and overwrite the cakephp .htaccess one of the foreign system. Now you should be able to access the foreign system just like you would access you cakephp app normally.
How to use CakePHP in the foreign system
Now open up the cakephp.php (the original index.php of app/webroot) and comment out the following lines at the end of the file like this:
{
}
else
{
/* $Dispatcher= new Dispatcher ();
$Dispatcher->dispatch($url);*/
}
Now you can include CakePHP via require_once() in the foreign system without a page beeing directly rendered. We want it like this because that makes it possible to do as many 'requestActions' inside drupal/wordpress/whatever as you need, which is essential to fully use cakephp in those apps. Now all you need is a function called requestCakePHP() in your foreign system in order to retrieve data produced by CakePHP. This is the one I've come up with:
{
// Sessions are done by Drupal, don't use CakePHP built in ones
if (!defined('AUTO_SESSION'))
define('AUTO_SESSION', false);
// Set the url parameter for cakephp
$_GET['url'] = $url;
require_once 'cakephp.php';
// We only need this to tell the name of the database we want to use,
// user/pass can be left blank for that reason.
if (!class_exists('DATABASE_CONFIG'))
{
eval("class DATABASE_CONFIG
{
var \$default = array('driver' => 'mysql',
'connect' => 'drupal_connection',
'host' => 'localhost',
'login' => 'root',
'password' => '',
'database' => 'drake',
'prefix' => '');
}");
}
// Fire up CakePHP and buffer results
ob_start();
$Dispatcher= new Dispatcher ();
$Dispatcher->dispatch($url);
return ob_get_clean();
}
function &drupal_connection()
{
// CakePHP expects this function to return a database connection, this is the one Drupal uses
global $active_db;
return $active_db;
}
Now most of this code just takes care of making sure that cakephp uses the same database as the foreign app and does NOT try to reconnect to it. The rest of the code should be fairly easy to understand.
The only thing you need to do now is to tell your foreign app to use requestCakePHP() for content retrieval when the system itself doesn't know how to handle a path. For Drupal this would be:
{
variable_set('site_404', 'drake');
}
function drake_menu($may_cache) {
$items = array();
$items[] = array('path' => 'drake',
'title' => t('Drake'), 'access' => true,
'callback' => 'requestCakePHP', 'type' => MENU_CALLBACK,
'callback arguments' => array($_GET['q']));
return $items;
}
That's it! And as you can see it's really something that should work with almost any other php application. The only thing you need to hope for is, that the other application doesn't provide functions with the same name as cakephp does inside basics.php. But since cakephp mostly uses classes for it's stuff luck should be on your side.
I hope I'll be able to release my Drake code (that's based on this new solution) soon, I'll write a blog post as soon as it's done.
--Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
Joel, thanks for your interest. The new Drake code will propably not contain too many things, since I've decided to start all over. But the difference will be that it will be way easier to implement, as well as more stable to run, and more flexible to use.
I hope I'll be done this weekend.
Very interesting stuff indeed Felix. Thanks for sharing! ;)
Hi Felix, thanks for sharing this. I'm reasonably new to Cake, and just decided to use acl for my project. I'll be sure to keep your article handy; It will sure spare me some pittfalls.
Hey marc, this sounds like this would be a response to my "CakePHP and Acl - Why is it so difficult?" post, so how does it happen to pop up here? ; ).
--Felix
Thank you for another informative posting. I just started with CakePHP a few weeks ago after several years of writing in PHP the "normal" way. Your postings have helped me solve several problems that have come up as a result of learning to do things the CakePHP way.
One question, though, when you say "Now open up the cakephp.php (the original index.php of app/webroot) and uncomment the following lines at the end of the file like this", should that actually say "comment out" instead of "uncomment"?
Thanks.
Dan.
Hi Dan, yes you are correct I ment to use "comment out" instead of "uncomment". English is not my first language (I'm German) so most of my posts could potentially contain more or less significant language mistakes in them. However, since you spotted this one for me, I corrected it in the post above - Thanks ; ).
Hi Felix,
I read about your Drake project, 2 months back. And I knew some of the developers from Drupal were also against that project because it was just a *redirection* trick according to them. Till then, I've been thinking about some solution to solve this. But WOW, You already posted it before I started learning Cake. I'm feeling much better now.
Thanks a lot. Max
Hey Felix,
I am able to come up with this: http://www.gigapromoters.com/blog/2007/01/28/joining-powers-of-two-great-systems-joomla-and-cakephp/
Thanks to you....
Hey Felix,
What’s up! Weird to see you outside of CakePHP google group
Have a question, what’s the status of this project? Can I extend it?
Using the same procedures I used for building Jake (following Max’s work, now available at http://dev.sypad.com/projects/jake) I just built a module for Drupal 5.1 that:
1. Same as Jake, allows your CakePHP application to run with or without Drupal, with no code modifications at either end.
2. Handles the possibility that Drupal & CakePHP may be using different databases.
3. Properly manages sessions (since Drupal may use a session handler that is not the same as Cake, plus Cake brings by default the AUTO_SESSION which would make your Cake application to complain when running inside Drupal): sessions are closed before running the application and restored afterwards, so your CakePHP application can use sessions at will, and Drupal doesn’t loose its session information.
4. No need to change any links or anything in your CakePHP application, the module automatically makes every link/AJAX resource to go through Drupal (*if* the CakePHP application is running on Drupal in the first place)
Well and a lot more things, so I was wondering how we can combine efforts.
Thanks mate! :)
PS: I posted this on an older blog post you had regarding the issue but it was throwing errors so I figured I tried here.
[...] this is just a quick announcement that a completely new Drake has has been released by Mariano Iglesias. For those of you who don’t remember: I used to have some fun with integrating CakePHP with other PHP applications around such as drupal which in term created a project called Drake. I only released a couple versions and by looking at Mariano documentation for Drakes none of them were as easy to set up. [...]
Well... that`s absolutely true, although cake saved our azes several times!, when deadlines knocked on the dor, and the day before nothing to show to our clients :O - when implementing it with common php apps, we haved bad $_SESSION issues, i will try this tricks to see what happens ...
... disabling the
Configure::write('Session.start', false);
not works/
well ... i done like here described, cake is killing my actual php session and i can`t do nothing about :(
i'm beginner using cakephp for final year project. i want to know where i can get installer of cakephp and how to install it?
i dont known anything about cakephp..
help me...
thanks..
@mimic, just download a stable build from:
www.cakephp.org
The installation process is nothing more than uploading the files and configurating some other settings (database, routes, etc.). You can find more about this at:
http://book.cakephp.org/view/32/installation
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
Thanks, Felix. I hope to play around with this some this weekend.
I'm looking forward to the update to your Drake code, also.
Thanks again!